DYNAMICS 365 CALL CUSTOM ACTION FROM JAVASCRIPT AND C#
In another post, we saw how to
call workflows from JavaScript and C#
.
In this post, I will show you how to call a Custom Action from JavaScript and C#.
For the sake of the post, I created a simple custom action against the Account entity with two input parameters and one output parameter.
This action will create a task record and set the Subject field to
- Task created by custom Action from JavaScript after calling the action from JavaScript
- Task created by custom Action from C# after calling the action from C#

Below is the end result after calling the action from JavaScript
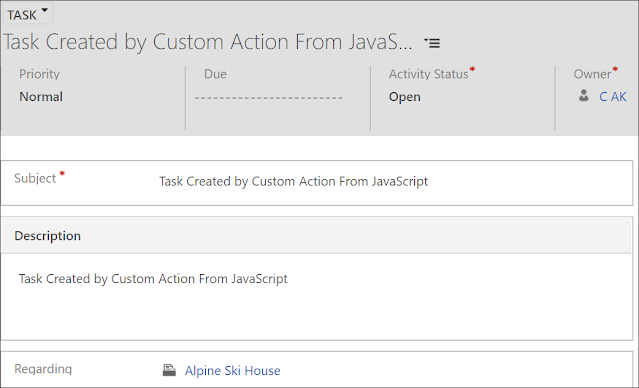
Below is the end result after calling the action from C#
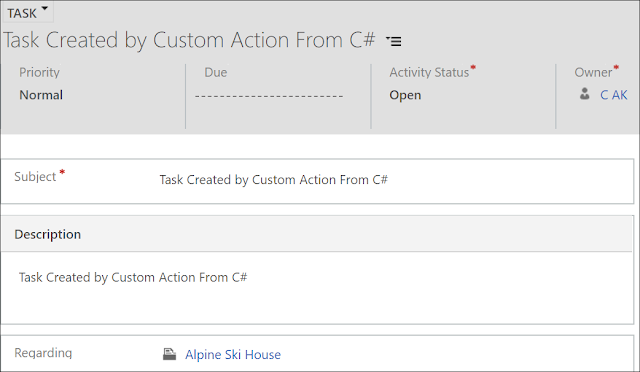
The below function calls the Custom Action in JavaScript and read its OutputParam
function callCustomAction(context) {var formContext = context.getFormContext();
var accountId = formContext.data.entity.getId().replace('{', '').replace('}', '');
var parameters = {};
var entity = {};
entity.id = accountId;
entity.entityType = "account";
parameters.entity = entity;
var inputparam1 = {};
inputparam1.accountid = accountId;
inputparam1["@odata.type"] = "Microsoft.Dynamics.CRM.account";
parameters.inputParam1 = inputparam1;
parameters.inputParam2 = 0;
var cak_CustomActionTestRequest = {
entity: parameters.entity,
inputParam1: parameters.inputParam1,
inputParam2: parameters.inputParam2,
getMetadata: function () {
return {
boundParameter: "entity",
parameterTypes: {
"entity": {
"typeName": "mscrm.account",
"structuralProperty": 5
},
"inputParam1": {
"typeName": "mscrm.account",
"structuralProperty": 5
},
"inputParam2": {
"typeName": "Edm.Int32",
"structuralProperty": 1
}
},
operationType: 0,
operationName: "cak_CustomActionTest"
};
}
};
Xrm.WebApi.online.execute(cak_CustomActionTestRequest).then(
function success(result) {
if (result.ok) {
var results = JSON.parse(result.responseText);
var outputParam = results.outputParam;
}
},
function (error) {
Xrm.Utility.alertDialog(error.message);
}
);
}
The below function calls the Custom Action in C# and read its OutputParam
public void ExecuteCustomAction(string actionName, Account targetEntity, Entity inputParam1Value, int inputParam2Value){
try
{
var testCustomAction = new OrganizationRequest()
{
RequestName = actionName,
Parameters = new ParameterCollection() {
new KeyValuePair<string, object>("inputParam1", inputParam1Value),
new KeyValuePair<string, object>("inputParam2", inputParam2Value)
}
};
testCustomAction["Target"] = new EntityReference(Account.EntityLogicalName, targetEntity.Id);
OrganizationResponse resp = AdminService.Execute(testCustomAction);
var outputParam = resp["outputParam"];
}
catch (InvalidPluginExecutionException ex)
{
throw new InvalidPluginExecutionException(ex.Message);
}
}
Bonus Tip:
- You can easily generate the code for calling your custom action in JavaScript by importing the CRMRESTBuilder solution and generate the request upon your need
Hope This Helps!
Comments
Post a Comment